Table of Contents
In this tutorial, I will take you through Primitive Data Types in Java. A programming language provides some predefined data types, which are known as built-in data types. There are basically eight built-in primitive data types in Java - int, char, byte, short, long, float, double and boolean. We will understand all the primitive data types in Java with the help of examples.
What is Primitive Data Type
A data type that consists of an atomic, indivisible value, and that is defined without the help of any other data types, is known as a primitive data type.
Primitive Data Types in Java
Also Read: Introduction to Java Programming - Java Development Kit(JDK) and Java Runtime Environment(JRE)
Java data types are divided into two categories: boolean
data type and numeric
data type. The numeric data type can be further subdivided into integral
and floating-point
types. All primitive data types and their categories are shown in below figure.
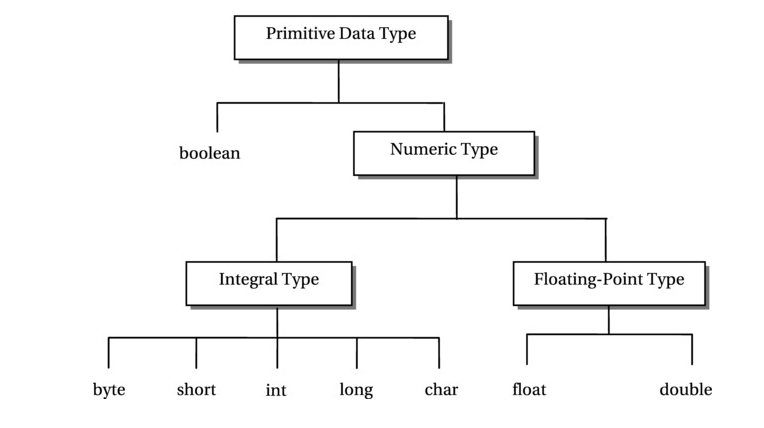
1. int datatype
- It is 32-bit Signed two's complement integer which can store value from -2,147,483,648 to 2,147,483,647.
- It take 4 bytes of memory to store the value. More on Oracle Official Documentation.
Example
In this example, we are declaring variable a
as primitive data type int
which stores value 5
.
[root@localhost ~]# vi example.java class example { public static void main(String[] args) { int a=5; System.out.println("Value of int type variable is: " + a); } }
Compile Your Program
[root@localhost ~]# javac example.java
Run Your Program
[root@localhost ~]# java example Value of int type variable is: 5
2. char datatype
- It is a Single 16-bit Unicode Character.
- It takes 2 bytes of Memory to store the value. More on Oracle Official Documentation.
Example
In this example, we are declaring variable a
as primitive data type char
which stores character 'a'
.
[root@localhost ~]# vi example.java class example { public static void main(String[] args) { char a='a'; System.out.println("Value of char type variable is: " + a); } }
Compile Your Program
[root@localhost ~]# javac example.java
Run Your Program
[root@localhost ~]# java example Value of char type variable is: a
3. byte datatype
- It is an 8-bit signed two's complement Integer which can store value from -128 to 127.
- It takes 1 byte of memory to store the value. More on Oracle Official Documentation.
Example
In this example, we are declaring variable a
as primitive data type byte
which stores value 114
.
[root@localhost ~]# vi example.java class example { public static void main(String[] args) { byte a=114; System.out.println("Value of byte type variable is: " + a); } }
Compile Your Program
[root@localhost ~]# javac example.java
Run Your Program
[root@localhost ~]# java example Value of byte type variable is: 114
4. short datatype
- It is a 16-bit signed two's complement Integer which can store value from -32,768 to 32,767.
- It takes 2 bytes of memory to store the value.
Example
In this example, we are declaring variable a
as primitive data type short
which stores value 9000
.
[root@localhost ~]# vi example.java class example { public static void main(String[] args) { short a=9000; System.out.println("Value of short type variable is: " + a); } }
Compile Your Program
[root@localhost ~]# javac example.java
Run Your Program
[root@localhost ~]# java example Value of short type variable is: 9000
5. long datatype
- It is a 64-bit two's complement integer which can store value from 9,223,372,036,854,775,808 to 9,223,372,036,854,775,807.
- It takes 8 bytes of memory to store the value.
- Value must end with 'L'.
Example
In this example, we are declaring variable a
as primitive data type long
which stores value 9000000000000000
.
[root@localhost ~]# vi example.java class example { public static void main(String[] args) { long a=9000000000000000L; System.out.println("Value of long type variable is: " + a); } }
Compile Your Program
[root@localhost ~]# javac example.java
Run Your Program
[root@localhost ~]# java example Value of long type variable is: 9000000000000000
6. float datatype
- It is a single-precision 32-bit IEEE 754 floating point which store fractional numbers.
- It takes 4 bytes of memory to store the value.
- Value must end with 'f'.
Example
In this example, we are declaring variable a
as primitive data type float
which stores value 5.988978
.
[root@localhost ~]# vi example.java class example { public static void main(String[] args) { float a=5.988978f; System.out.println("Value of float type variable is: " + a); } }
Compile Your Program
[root@localhost ~]# javac example.java
Run Your Program
[root@localhost ~]# java example Value of float type variable is: 5.988978
7. double datatype
- It is a single-precision 64-bit IEEE 754 floating point which store fractional numbers.
- It takes 8 bytes of memory to store the value.
Example
In this example, we are declaring variable a
as primitive data type double
which stores value 5.98657667998978
.
[root@localhost ~]# vi example.java class example { public static void main(String[] args) { double a=5.98657667998978d; System.out.println("Value of double type variable is: " + a); } }
Compile Your Program
[root@localhost ~]# javac example.java
Run Your Program
[root@localhost ~]# java example Value of double type variable is: 5.98657667998978
8. boolean datatype
- It stores only two possible values - true or false.
- It only requires 1 bit of memory to store the value.
Example
In this example, we are declaring variable a
as primitive data type boolean
which stores value true
.
[root@localhost ~]# vi example.java class example { public static void main(String[] args) { boolean a=true; System.out.println("Value of boolean type variable is: " + a); } }
Compile Your Program
[root@localhost ~]# javac example.java
Run Your Program
[root@localhost ~]# java example Value of boolean type variable is: true
Popular Recommendations:-
Step by Step Guide to Install Apache 2.4.6 Web Server on RHEL/CentOS 7
How to Install MariaDB 5.5 Server on RHEL/CentOS 7 Linux with Easy Steps
6 Simple Steps to Change/Reset MariaDB root password on RHEL/CentOS 7/8
Best Steps to Install Java on RHEL 8/CentOS 8
5 Examples to Turn Off SELinux Temporarily or Permanently on RHEL 8/CentOS 8
Best Explanation of Wrapper Classes in Java: Autoboxing and Unboxing with Examples
5 Best Ways to Become root user or Superuser in Linux (RHEL/CentOS/Ubuntu)